why people delete the recycle bin
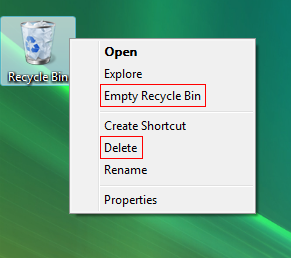
I would have thought that using "Remove Recycle Bin" or even "Delete Recycle Bin" rather than "Delete" would have been a better option.
I guess that MS didn't pass round the "Don't make me think" book.
// get the date time for the report
DateTime dt = session.createDateTime("Today");
dt.setNow();
// the generic data object
PdfData data = new PdfData();
// where to put the file
PdfDocumentConfig config = new PdfDocumentConfig("Text",
"ViewByTitle.pdf", "c:\\pdfs");
/*
* Set up some of the styles that
* we will use in the pdf document.
*/
// the style for the database and view title
Style styleViewTitle = new Style("viewTitle", Style.TYPEFACE_HELVETICA,
18);
styleViewTitle.setDecoration(Style.DECORATION_BOLD);
styleViewTitle.setAlign(Style.ALIGN_CENTER);
styleViewTitle.setBackgroundColour(232, 232, 232);
styleViewTitle.setColor(0, 94, 187);
// the style for the date stamp
Style styleDate = new Style("date", Style.TYPEFACE_HELVETICA, 10);
styleDate.setAlign(Style.ALIGN_RIGHT);
styleDate.setBackgroundColour(Color.RED);
// the style for the view column titles
Style styleColumnTitle = new Style("columnsTitle",
Style.TYPEFACE_HELVETICA, 12);
styleColumnTitle.setDecoration(Style.DECORATION_BOLD);
styleColumnTitle.setBackgroundColour(232, 232, 232);
styleColumnTitle.setColor(0, 94, 187);
// the style for the column categories
Style styleColumnCat = new Style("columnsCat", Style.TYPEFACE_HELVETICA,
12);
styleColumnCat.setDecoration(Style.DECORATION_BOLD);
styleColumnCat.setBackgroundColour(247, 247, 247);
styleColumnCat.setColor(255, 153, 0);
// the default style for the view data
Style styleViewDefault = new Style("default", Style.TYPEFACE_HELVETICA,
10);
/*
* set header image - used the image from the superNTF nsf
*/
HeaderImage headerImage = new HeaderImage();
headerImage.addImage("c:\\pdfs\\BrandBanner.gif");
headerImage.setImageScale(HeaderImage.IMAGE_NO_SCALE, false);
// create a header to write the date into
HeaderTwoColumns header = new HeaderTwoColumns(50, 50);
header.setLeftColumn(new CellElement("", styleDate));
header.setRightColumn(new CellElement(dt.getDateOnly(), styleDate));
header.setStyle(styleDate);
header.setHeight(100);
// create a footer
FooterTwoColumns footer = new FooterTwoColumns(50, 50);
footer.setLeftColumn(new CellElement(
"http://palmerweb.blogspot.com - http://www.scius.com.au"));
footer.setRightColumn(new CellElement("", styleDate));
footer.setStyle(styleViewDefault);
footer.setPageNum(new PageNumbersElement(styleDate));
footer.includePageNumbers(true,
FooterTwoColumns.PAGE_NUMBERS_RIGHT);
// getting the notes handles
AgentContext ctx = session.getAgentContext();
Database db = ctx.getCurrentDatabase();
// this is the view
View viewMembers = db.getView("vw-docsbytitle");
// read the columns to get the report headings
Vector cols = viewMembers.getColumns();
// filter out the columns that we are not interested in
// such as icons, hidden, total details and categories
Vector colsToRead = new Vector();
for (int x = 0; x < cols.size(); x++) {
ViewColumn col = (ViewColumn) cols.get(x);
if (col.isIcon() == false && col.isCategory() == false
&& col.isHidden() == false
&& col.isHideDetail() == false) {
colsToRead.add(col);
}
}
// create a table based on the number of columns
TableElement tb = new TableElement(colsToRead.size());
/*
* create the heading (repeating) rows for the table.
*/
// database and view title
RowElement rw = new RowElement();
CellElement title = new CellElement(db.getTitle() + " - "
+ viewMembers.getName() + "\n\n", styleViewTitle);
title.setCellspan((colsToRead.size()));
rw.addCell(title);
rw.setTableHeading(true);
tb.AddRow(rw);
// column headings
rw = new RowElement();
for (int x = 0; x < colsToRead.size(); x++) {
ViewColumn col = (ViewColumn) colsToRead.get(x);
rw
.addCell(new CellElement(col.getTitle() + "\n\n",
styleColumnTitle));
}
rw.setTableHeading(true);
tb.AddRow(rw);
/*
* populate the table with the view data
*/
ViewNavigator viewNav = viewMembers.createViewNav();
ViewEntry rowData = viewNav.getFirst();
while (!(rowData == null)) {
rw = new RowElement();
Vector viewCol = rowData.getColumnValues();
// check to see if this is a category
if (rowData.isCategory()) {
CellElement catCell = new CellElement("\n"
+ viewCol.get(1).toString() + "\n\n", styleColumnCat);
catCell.setCellspan(colsToRead.size());
rw.addCell(catCell);
} else if (rowData.isDocument()) {
for (int x = 0; x < colsToRead.size(); x++) {
ViewColumn col = (ViewColumn) colsToRead.get(x);
int pos = col.getPosition() - 1;
rw.addCell(new CellElement(viewCol.get(pos).toString(),
styleViewDefault));
}
}
tb.AddRow(rw);
rowData = viewNav.getNext(rowData);
}
// add the table to the data object
data.add(tb);
// overide the default layout
LayoutElement layout = new LayoutElement(LayoutElement.PAGESIZE_A4,
LayoutElement.ORIENTATION_LANDSCAPE, 10, 10, 10, 10, 40, 50);
// add the image to the layout
layout.setHeaderImage(headerImage);
layout.setFooter(footer);
layout.setHeader(header);
// configure with the config, data and layout
PdfDocument pdf = new PdfDocument(config, data, layout);
// go
pdf.createDocument();
Watch the movie to see how to create a pdf of a view in 60 seconds.
I've produced a movie to demonstrate how easy it is to write an agent that creates a pdf of a view using my new library. I chose SuperNTF as it was the first database that had a flat view and a selection of documents. I copied and pasted enough entries to give me two pages so that you can see repeating headers. It's not quite as entertaining as 'Gone in 60 seconds' but does show you how quickly you can implement this in your own databases.
Below is the example code from the movie that simply dumps the content of a designated flat view to a table in a pdf. I've updated the library to Beta 7 to contain a minor enhancement to allow you to specify rows that are designated as headers. These header rows (yes you can specify more than one) are repeated on each page as the content forces a page break. Again, most of the heavy lifting is done by iText.
The Code
// the generic data object
PdfData data = new PdfData();
// where to put the file
PdfDocumentConfig config = new PdfDocumentConfig("Text",
"agent-test.pdf", "c:\\temp");
// getting the notes handles
AgentContext ctx = session.getAgentContext();
Database db = ctx.getCurrentDatabase();
// this is the view
View viewMembers = db.getView(" - insert your view alias here - ");
// get the column names for a header
Vector cols = viewMembers.getColumnNames();
// create a table based on the number of columns
TableElement tb = new TableElement(cols.size());
// start populating with the column names
RowElement rw = new RowElement();
for (int x = 0; x < cols.size(); x++) {
rw.addCell(new CellElement(cols.get(x).toString()));
}
// mark this as a table header to be repeated
rw.setTableHeading(true);
tb.AddRow(rw);
// start populating the table with the view data
ViewNavigator viewNav = viewMembers.createViewNav();
ViewEntry rowData = viewNav.getFirst();
while (!(rowData == null)) {
rw = new RowElement();
Vector viewCol = rowData.getColumnValues();
for (int y = 0; y < viewCol.size(); y++) {
rw.addCell(new CellElement(viewCol.get(y).toString()));
}
tb.AddRow(rw);
rowData = viewNav.getNext(rowData);
}
// add the table to the data object
data.add(tb);
// overide the default layout
LayoutElement layout =
new LayoutElement(LayoutElement.PAGESIZE_A4,
LayoutElement.ORIENTATION_LANDSCAPE,
10, 10, 10, 10, 10, 10);
// configure with the config, data and layout
PdfDocument pdf = new PdfDocument(config, data, layout);
// go
pdf.createDocument();
} catch (Exception e) {
e.printStackTrace();
} finally {
}
I've seen the move so how can I use this ?
1. Download Beta 7, and extract the updated jar file to a directory c:\lib
2. Download The JavaAgentPdf code
3. Create a new Java agent in your database, add the iText-2.0.8 jar and the new scius-pdfdoc.beta7.jar
3. Replace the JavaAgent code with the downloaded version and update the view name, pdf filename and pdf directory.
Enhancing the example further.
To keep this example short and under 30 lines, I have skipped of some of the things that you should add in for a production implementation, such as dealing with exceptions and checking that the domino objects are not null. However, for a proof-of-concept - it might be adequate. You would also need to write a few more lines to account for a categorised views, hidden columns and possibly the final enhancement would be to make the report look nice with some styles.
Stay tuned because the next post might have an example that produces a pdf that is a bit nicer on the eyes.
Update : The link to the zip file has changed. I found a bug with Beta7 and ParagraphElements.
/*
* Create a config object to contain file location and
* some meta data settings
*/
config = new PdfDocumentConfig("Example 3","example3.pdf","examples");
config.setReportSubject("Example 3 - Image banner");
config.setReportAuthor("Scius and iText");
/*
* Creating a header image
*/
headerImage = new HeaderImage();
headerImage.addImage(new String("examples/top.jpg"));
headerImage.setImageScale(HeaderImage.IMAGE_PAGE_SCALE, true);
/*
* Create a layout, size, orientation, margins, header and footer
*/
layout = new LayoutElement(
LayoutElement.PAGESIZE_A4,
LayoutElement.ORIENTATION_PORTRAIT,
20,20,10,10,100,100
);
// add the image to the layout
layout.setHeaderImage(headerImage);
/*
* Create the PDF content, the data written to the PDF
*/
data = new PdfData();
data.add(new ParagraphElement("Hello World"));
/*
* Create a instance of the PDF Document writer, using
* the config, data and layout
*/
pdf = new PdfDocument(config, data, layout);
/*
* generate the document
*/
pdf.createDocument();
/*
* Create a config object to contain file location and
* some meta data settings.
*/
config = new PdfDocumentConfig("Example 5","example5.pdf","examples");
config.setReportSubject("Example 4 - Tables");
config.setReportAuthor("Scius and iText");
/*
* Creating a header image.
*/
headerImage = new HeaderImage();
headerImage.addImage(new String("examples/top.jpg"));
headerImage.setImageScale(HeaderImage.IMAGE_PAGE_SCALE, true);
/*
* Set the default style and page numbers style.
*/
Style defaultStyle = new Style("default",Style.TYPEFACE_TIMES_ROMAN,15);
// styles for the table
Style tableStyle = new Style("default", Style.TYPEFACE_HELVETICA, 10);
tableStyle.setColor(Color.BLUE);
Style tableHeaderStyle = new Style("default", Style.TYPEFACE_HELVETICA, 10);
tableHeaderStyle.setDecoration(Style.DECORATION_BOLD);
tableHeaderStyle.setBackgroundColour(192, 192, 192);
Style stylePageNumbers = new Style("pagenumbers", Style.TYPEFACE_TIMES_ROMAN, 15);
stylePageNumbers.setAlign(Style.ALIGN_RIGHT);
/*
* Create a two column footer.
*/
footer = new FooterTwoColumns(50,50);
footer.setLeftColumn(new CellElement("Example 4"));
footer.setRightColumn(new CellElement("",stylePageNumbers));
footer.setStyle(defaultStyle);
footer.setPageNum(new PageNumbersElement(stylePageNumbers));
footer.includePageNumbers(true,FooterTwoColumns.PAGE_NUMBERS_RIGHT);
/*
* Create a layout, size, orientation, margins, header and footer
*/
layout = new LayoutElement(
LayoutElement.PAGESIZE_A4,
LayoutElement.ORIENTATION_PORTRAIT,
20,20,10,10,100,100
);
layout.setHeaderImage(headerImage);
layout.setFooter(footer);
/*
* Create the PDF content, the data written to the PDF.
*/
data = new PdfData();
data.add(new ParagraphElement("Hello World", defaultStyle));
data.add(new ParagraphElement("table of authors\n\n", defaultStyle));
// add tabular information
TableElement table = new TableElement(3);
// define the column widths
float[] wd = {50f, 25f,25f};
table.setColumnWidths(wd);
// start adding data
RowElement row = new RowElement();
row.addCell(new CellElement("Author",tableHeaderStyle));
CellElement ce = new CellElement("Book Details",tableHeaderStyle);
ce.setCellspan(2);
row.addCell(ce);
table.AddRow(row);
RowElement row2 = new RowElement();
row2.addCell(new CellElement("Bruno Lowgie", tableStyle));
row2.addCell(new CellElement("iText in Action", tableStyle));
row2.addCell(new CellElement("http://www.lowagie.com/iText",tableStyle));
table.AddRow(row2);
RowElement row3 = new RowElement();
row3.addCell(new CellElement("Bruce Eckel", tableStyle));
row3.addCell(new CellElement("Thinking in Java", tableStyle));
row3.addCell(new CellElement("http://mindview.net/Books/TIJ4",tableStyle));
table.AddRow(row3);
// add the table to the data transfer object
data.add(table);
/*
* Create a instance of the PDF Document writer, using
* the config, data and layout.
*/
pdf = new PdfDocument(config, data, layout);
/*
* creates the pdf document file.
*/
pdf.createDocument();